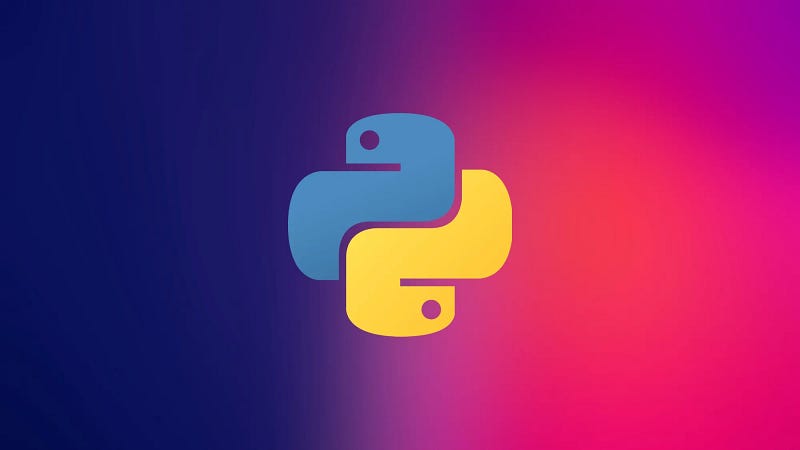
PYTHON — Analyzing Ratios with Python
The most dangerous phrase in the language is, ‘We’ve always done it this way.’ — Grace Hopper
Insights in this article were refined using prompt engineering methods.
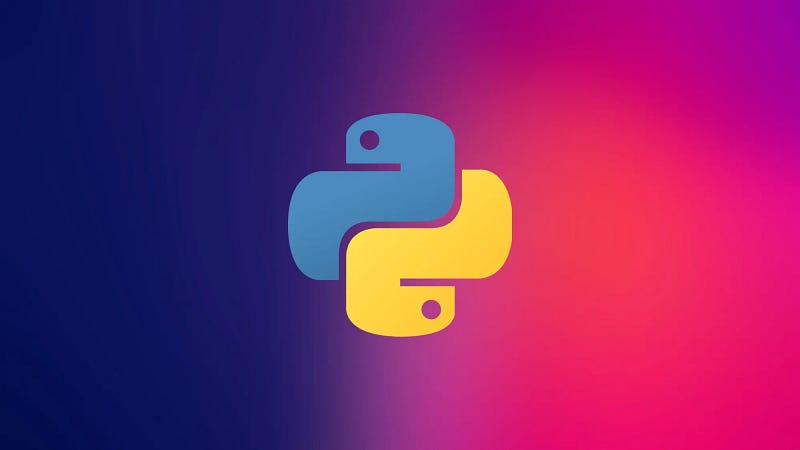
PYTHON — Types Of Python Methods A Recap And Review
# Analyzing Ratios with Python
In this tutorial, we will explore the process of determining ratios using Python. Ratios are a fundamental concept in mathematics and are widely used in various fields such as finance, engineering, and data analysis. We will cover the practical applications of determining ratios, the necessary Python libraries, and step-by-step guidance on creating a project related to determining ratios using Python.
Practical Applications of Determining Ratios
Determining ratios is essential for comparing quantities, making predictions, and analyzing trends. Some practical applications include:
- Financial analysis: Calculating financial ratios such as debt-to-equity ratio, price-earnings ratio, etc.
- Engineering: Analyzing gear ratios, voltage ratios, etc.
- Data analysis: Calculating proportions, growth rates, and other statistical ratios.
Required Python Libraries
For this project, we will use the following Python libraries:
- Pandas: Essential for data manipulation and analysis.
- NumPy: For numerical operations and array manipulations.
- Matplotlib: For visualizing data and creating plots.
- Seaborn: An additional library for statistical data visualization.
Make sure you have Python and pip (Python’s package installer) installed on your system. Let’s start by setting up the project environment and installing the required libraries.
Step 1: Setting Up the Project Environment
Open your terminal or command prompt and create a new directory for your project. Then, navigate into the newly created directory.
mkdir determining-ratios-project
cd determining-ratios-project
Now, let’s create a virtual environment to isolate our project dependencies.
python -m venv env
Activate the virtual environment:
- On Windows:
env\Scripts\activate
- On macOS and Linux:
source env/bin/activate
Next, install the required Python libraries using pip:
pip install pandas numpy matplotlib seaborn
With the environment set up and the necessary libraries installed, we can now begin the foundational steps of the project.
Step 2: Foundational Steps
Importing Required Libraries
Create a new Python file, such as ratio_analysis.py
, and import the required libraries.
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
Loading and Preparing Data
For this example, we will use a sample dataset to demonstrate ratio analysis. You can use your own dataset or a publicly available dataset.
Assuming you have a CSV file named financial_data.csv
, load the data using Pandas.
# Load the data into a DataFrame
financial_data = pd.read_csv('financial_data.csv')
# Display the first few rows of the DataFrame
print(financial_data.head())
Calculating Ratios
Now, we’ll calculate some financial ratios using the loaded data. Here’s an example of calculating the debt-to-equity ratio:
# Calculate debt-to-equity ratio
financial_data['debt_to_equity_ratio'] = financial_data['total_debt'] / financial_data['shareholders_equity']
# Display the updated DataFrame with the new ratio column
print(financial_data.head())
Visualizing Ratios
To visualize the calculated ratios, we can create a bar plot using Matplotlib and Seaborn.
# Create a bar plot for debt-to-equity ratio
plt.figure(figsize=(10, 6))
sns.barplot(x='company_name', y='debt_to_equity_ratio', data=financial_data)
plt.title('Debt-to-Equity Ratio by Company')
plt.xlabel('Company')
plt.ylabel('Debt-to-Equity Ratio')
plt.xticks(rotation=45)
plt.show()
Conclusion
In this tutorial, we’ve set up a project environment for determining ratios using Python, loaded and prepared data, calculated ratios, and visualized the results. We also explored practical applications of determining ratios and the necessary Python libraries for the project.
Best practices for determining ratios in Python include using efficient data structures, optimizing algorithms for ratio calculations, and ensuring data integrity throughout the analysis process.
To further expand this project, you can consider implementing additional ratio calculations, integrating external data sources, or building a web application for interactive ratio analysis.
I hope this tutorial provides a solid foundation for your journey in determining ratios using Python! Happy coding!
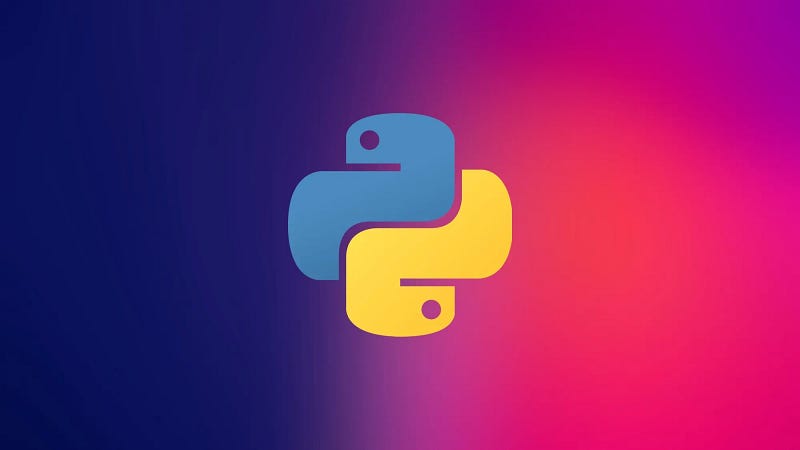