Understanding Literals in Java
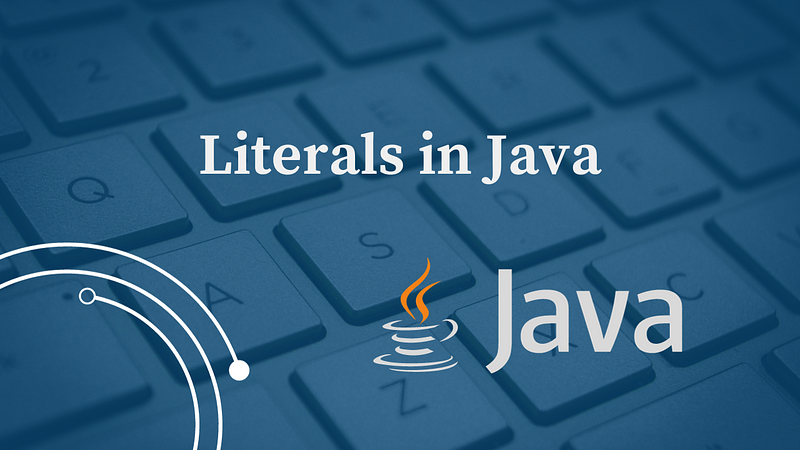
Java has undergone significant evolution since its inception. Each new version brings improvements and new features, but one concept that has remained fundamental throughout Java’s history is the “literal”.
In the Java programming language, literals are a fundamental concept that every developer comes across. These building blocks represent fixed values. Developers can assign them to variables and manipulate during program execution. They form the foundation of any Java application. In this article, we’ll explore what literals are, why they are essential, and why Java doesn’t need a new keyword to handle them.
If you don’t have a medium membership, you can use this link to reach the article without a paywall.
What are Literals?
Literals are simply constants that represent fixed values in your code. They are a direct and efficient way to specify a value. Think of them as direct representations of numbers, characters, booleans, and strings.
When you write a number like 42
, a character like 'A'
, a boolean like true
, or a string like "Hello"
, you're using a literal. In Java, literals serve as a direct way to assign values to variables. They are an integral part of the language’s syntax.
Types of Literals in Java
Java supports several types of literals:
Numeric Literals
Numeric literals represent numeric values. Java supports various types of numeric literals, including:
- Integer Literals: These are whole numbers, like 42 or -17. Typically, they express these values without a fractional or decimal part.
- Floating-Point Literals: These are numbers with a fractional or decimal part. For example, 3.14 or -0.01. Java allows both single-precision (float) and double-precision (double) floating-point literals.
- Scientific Notation Literals: In scientific notation, you can represent numbers with an exponent. For instance, 2.5E3 is equivalent to 2500.
Character and String Literals
You can represent characters and strings using literals in Java. Here are some examples:
- Character Literals: We enclose a character literal, like ‘A’, ‘b’, or ‘1’ in single quotes. Additionally, we can express special characters, such as ‘\n’ or ‘\t’, as literals.
- String Literals: Strings are sequences of characters enclosed in double quotes, like “Hello, World!” or “Java Programming.”
Boolean Literals
Java uses boolean literals to represent true
or false
values. Developers frequently use them in conditional statements, such as if
or while
.
Null Literal
The null
keyword is a special literal in Java, indicating the absence of a value.
Examples of Literals
Let’s explore some examples of how developers use literals in Java:
int age = 30; // 30 is an integer literal
byte noOfGroups = 100; // 100 is byte literal
short noOfStudents = 10000; // 10000 is short literal
double price = 19.99; // 19.99 is a floating-point literal
char grade = 'A'; // 'A' is a character literal
String greeting = "Hi"; // "Hi" is a string literal
boolean isJavaFun = true; // true is a boolean literal
Object obj = null; // null is a null literal
The Power of Literals
Literals play a crucial role in Java, and they bring several advantages to the language.
1. Readability
One of the primary advantages of using literals is improving code readability. Because when you see a numeric or string literal in the source code, you instantly understand the value being assigned to a variable or used in an expression. This readability simplifies code comprehension and maintenance, making it easier for developers to work with Java.
For example, consider the following code snippet:
int numberOfStudents = 50;
In this context, it’s clear that the variable numberOfStudents
receives the literal value of 50. This simplicity in code representation helps in reducing errors and enhancing the overall development process.
2. Expressiveness
Literals enhance the expressiveness of Java code. They provide a concise and clear way to express data. For instance, when dealing with constants, you can use a final
modifier with a literal to make your intention clear:
final int DAYS_IN_A_WEEK = 7;
In this case, it’s evident that DAYS_IN_A_WEEK
should not change its value, as it is marked as final
. Using a literal in this context reinforces the constant nature of the variable.
3. Efficiency
Literals offer efficiency in code execution. Since they represent fixed values, the compiler can optimize their usage, resulting in faster and more efficient code. This is particularly beneficial when working with primitive data types like int
, double
, or char
.
The ‘new’ Keyword in Java
Before we understand why we don’t need the new
keyword for literals, let's clarify what new
does. Developers use the new
keyword to create new objects in Java. It initializes a new instance of a class and returns a reference to that memory. For instance, to create a new instance of a String
object, you would typically write:
String example = new String("This is a string.");
However, for creating literals, we don’t use new
. Let's see why.
Why Don’t We Need the ‘new’ Keyword for Literals?
The primary reason is that literals represent constant, immutable values directly in the code. They are intrinsically fixed and unchangeable. Therefore there is no need to allocate separate memory for them each time they are used.
Efficiency and Performance
Java has a neat feature called the “constant pool.” This is a special memory space where Java stores literals. For instance, every time you use the integer 42
in your code, Java doesn't create a new memory allocation. Instead, it refers to the 42
that exists in the constant pool.
This system is much more efficient than using new
, which would create a new memory space every time. For literals, which are often used repeatedly, this would be wasteful and could significantly impact performance.
Immutable Nature
Strings are a great example to explain this concept. In Java, strings are immutable; once you create a string, you cannot change it. Because of this, it makes sense to use the string literal notation, which uses the constant pool. If you use the new
keyword, you create a new string object, not a literal, and the constant pool does not store it.
Consider these two lines of code:
String hello = "Hello";
String anotherHello = new String("Hello");
In the first line, hello
refers to a string literal that might already be in the constant pool. In the second line, anotherHello
forces the creation of a new string object, even though it represents the same sequence of characters as the literal.
Special Case: Integer Literals and Interning
Integer literals in Java are a special case. The Java specification states that it caches and reuses integers between -128 and 127, a process known as interning
. So, when you use an integer literal within this range, you’re likely getting a cached value, similar to how string literals work.
If you are interested about interned strings
When to Use Literals and ‘new’
Understanding when to use literals and when to use new
is crucial:
- Use literals when dealing with fixed, immutable values. It’s quick, efficient, and easy to read.
- Use
new
when you need to create new instances of objects where each instance is distinct, or when you're working with classes that are mutable.
Practical Example : String Literals vs. String Objects
To solidify our understanding, let’s look at a practical example.
String str1 = "Java"; // Uses the string literal
String str2 = "Java"; // Reuses the string literal from the constant pool
String str3 = new String("Java"); // Creates a new string object, not from the constant pool
System.out.println(str1 == str2); // true, because str1 and str2 refer to the same literal
System.out.println(str1 == str3); // false, because str3 refers to a different object
This example demonstrates the difference between using literals and the new
keyword. str1
and str2
point to the same location in memory, while str3
points to a newly allocated space.
Literals in Java provide a streamlined, efficient way to use constant values in your programs. The design of Java’s memory management for literals through the constant pool offers performance benefits and ease of use. The ‘new’ keyword, while essential for creating new object instances, is unnecessary for literals because of their immutable and constant nature. As a developer, recognizing when to use literals versus new
can lead to cleaner, more efficient code.
👏 Thank You for Reading!
👨💼 I appreciate your time and hope you found this story insightful. If you enjoyed it, don’t forget to show your appreciation by clapping 👏 for the hard work!
📰 Keep the Knowledge Flowing by Sharing the Article!
✍ Feel free to share your feedback or opinions about the story. Your input helps me improve and create more valuable content for you.
✌ Stay Connected! 🚀 For more engaging articles, make sure to follow me on social media:
🔍 Explore More! 📖 Dive into a treasure trove of knowledge at Codimis. There’s always more to learn, and we’re here to help you on your journey of discovery.