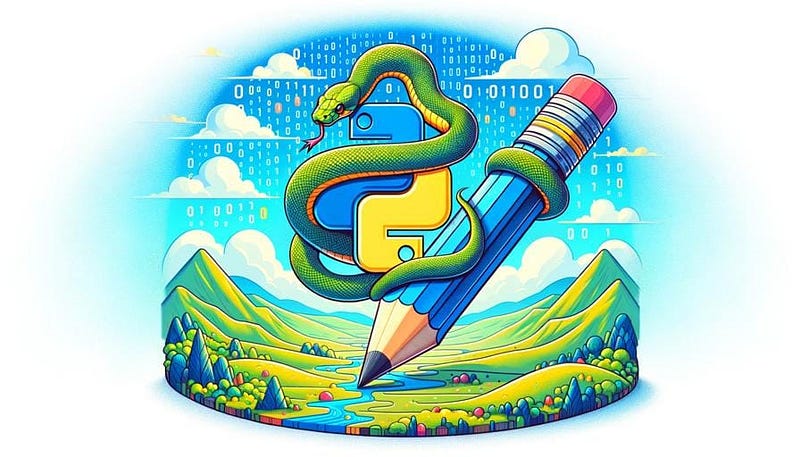
Type Promotion in Python: A Simplified Approach for Beginners
Are you ready to become a Python pro? Let’s start with understanding type promotion in functions, an essential skill for writing clean and error-free code.
Understanding Python’s Type Promotion in Functions
Hey there, Python lovers! 🐍 Today, we’re tackling an intriguing aspect of Python programming: type promotion in functions. It’s a cool concept, but it can be a bit tricky, especially for those just starting out. So, let’s break it down.
Consider this situation: You have a function that multiplies two numbers. These numbers can be either int
or float
. How do you make sure your function returns the right type based on the inputs? 🤔
def mul(a: int | float, b: int | float): # return type?
return a * b
Here’s where the challenge comes in. If both a
and b
are integers, the function should return an integer. Otherwise, it should return a float. The big question is, how do you annotate the return type in Python to handle this?
One approach is using @overload
from the typing
module. But, as you'll see, it can get a bit wordy.
So, back to our mul
function. The @overload
decorator lets you define multiple versions of a function, each with different type signatures. Here's how it looks:
from typing import overload
@overload
def mul(a: int, b: int) -> int: ...
@overload
def mul(a: float, b: int | float) -> float: ...
@overload
def mul(a: int | float, b: float) -> float: ...
def mul(a, b):
return a * b
With this setup, Python understands the specific return type based on the types of the inputs. But it’s quite verbose, right? Let’s admit it, this isn’t the most elegant solution. 😕
What if we could have something simpler? Like in C++, where you can use techniques like SFINAE for type promotion. Unfortunately, Python doesn’t have a built-in feature exactly like that. However, we’re not out of options! 🚀
One technique we can explore is the use of generics with TypeVar
from Python's typing
module. This allows you to create a type variable that can be either an int
or a float
. Here's an example:
from typing import TypeVar
T1 = TypeVar('T1', int, float)
T2 = TypeVar('T2', int, float)
def mul(a: T1, b: T2) -> ???
return a * b
The tricky part is annotating the return type. A custom function for type promotion might seem like a solution, but remember, Python’s type system doesn’t work exactly like C++’s. The goal is to find a balance between accuracy and simplicity. So, let’s keep exploring other possibilities. 🌟
Wrapping Up: Python Type Hints and FAQs
Alright, folks! We’ve had a deep look into Python type hints and how they can help in specifying types in your functions. Let’s wrap it up with some key takeaways and answer some common questions you might have.
References:
Python 3.12 Type Hints Documentation
FAQs:
Q: What are Python type hints?
A: Type hints are annotations that you can add to Python code to specify what type of data each variable should hold. They help in making your code more readable and easier for others to understand.
Q: How do I use type hints in Python?
A: You can use type hints by adding annotations next to variables and function parameters. For example, to hint that a function parameter should be an integer, you’d write: def my_function(param: int):
.
Q: Are type hints mandatory in Python?
A: No, they’re not mandatory but highly recommended. They make your code clearer and can assist in debugging and development processes.
Originally published on HackingWithCode.com.