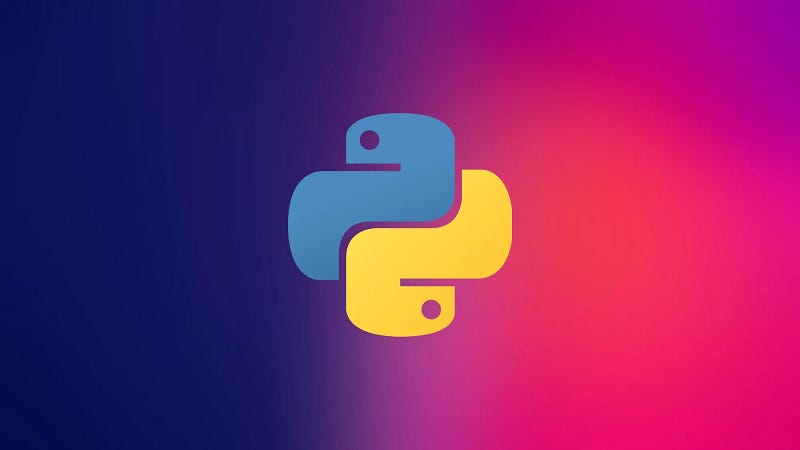
PYTHON — Staying On The Screen In Python
Real artists ship. — Steve Jobs
Insights in this article were refined using prompt engineering methods.
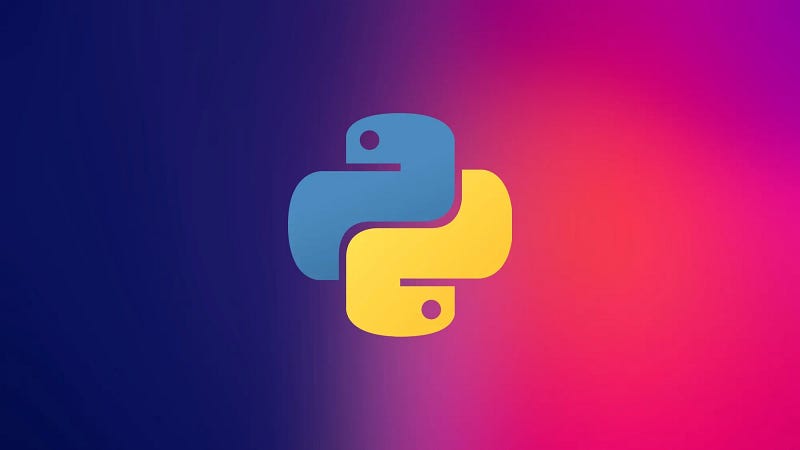
PYTHON — Python Fibonacci Sequence Tutorial
>
### Staying on the Screen in Pygame with Python
In this lesson, we’ll learn how to fix the issue of staying on the screen. If you’ve been following along, you may have noticed a couple of small problems with your game. The first of which is that the player rectangle can move off the screen. The other issue is the player moving very fast when a key is held down.
To address the problem of staying on the screen, you need to add some logic to detect if the player is going to move off the screen. To do this, you can check whether the player’s coordinates have moved beyond the screen’s boundary. If so, you instruct the program to move the player back to the edge.
# Move the player sprite based on user keypresses
def update(self, pressed_keys):
if pressed_keys[K_UP]:
self.rect.move_ip(0, -5)
if pressed_keys[K_DOWN]:
self.rect.move_ip(0, 5)
if pressed_keys[K_LEFT]:
self.rect.move_ip(-5, 0)
if pressed_keys[K_RIGHT]:
self.rect.move_ip(5, 0)
# Keep player on the screen
if self.rect.left < 0:
self.rect.left = 0
if self.rect.right > SCREEN_WIDTH:
self.rect.right = SCREEN_WIDTH
if self.rect.top <= 0:
self.rect.top = 0
if self.rect.bottom >= SCREEN_HEIGHT:
self.rect.bottom = SCREEN_HEIGHT
In this code snippet, we have a method called update
which takes the pressed_keys
as input. Depending on the keys pressed, the player's position is updated. Additionally, we have added logic to ensure that the player stays within the boundaries of the screen.
By checking the player’s position against the screen boundaries, we ensure that the player remains visible and doesn’t move off the edge of the screen. This enhances the user experience and prevents any unexpected behavior in the game.
After adding these lines of code, you should see that the player is now constrained to the screen. Try moving the player around and observe how it stays within the boundaries.
In the next lesson, we will start setting up enemies for the game. Stay tuned for more updates!
I hope you found this lesson helpful. If you have any questions or feedback, feel free to leave a comment below. Happy coding!
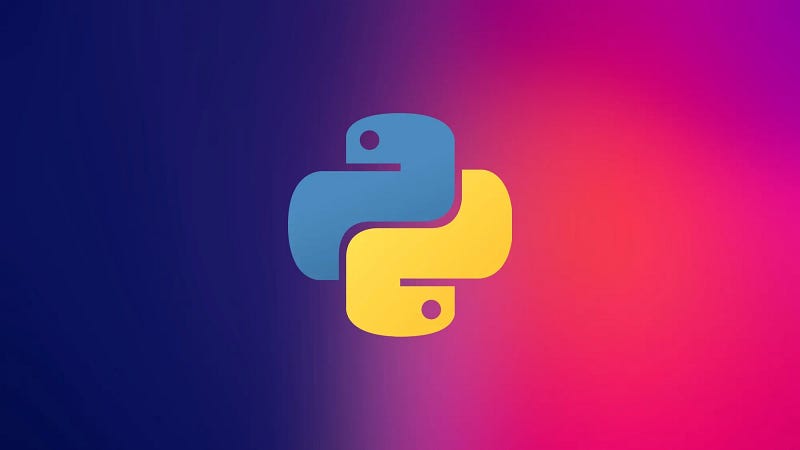