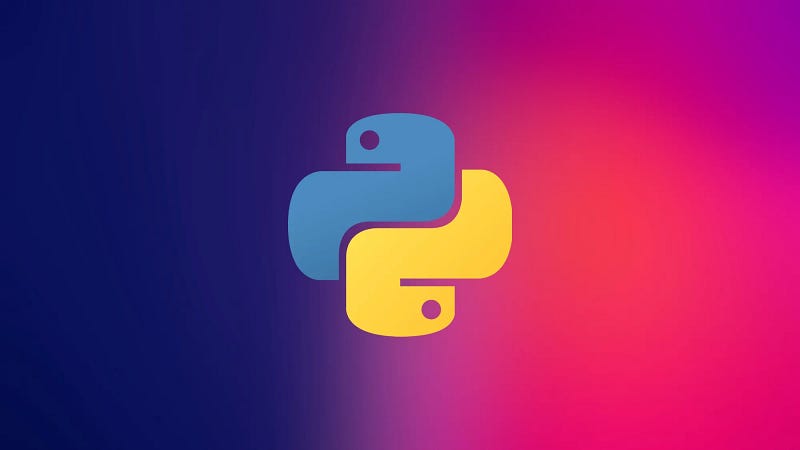
PYTHON — Managing Contexts With Patch In Python
Measuring programming progress by lines of code is like measuring aircraft building progress by weight. — Bill Gates
Insights in this article were refined using prompt engineering methods.
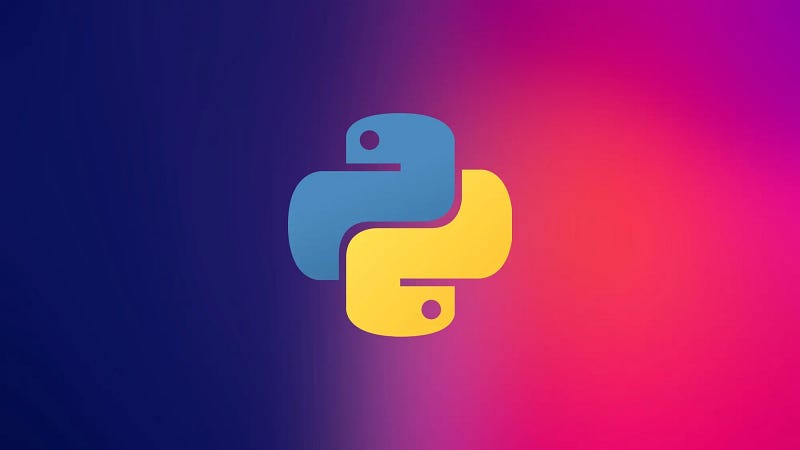
PYTHON — Python Fibonacci Sequence Tutorial
>
# Managing Contexts with Patch in Python
The patch()
function in the unittest.mock
module allows you to temporarily replace an object in a test. It can be used as a decorator or as a context manager. In this tutorial, you will learn how to use patch()
as a context manager to manage contexts in Python.
Using patch() as a Context Manager
When using patch()
as a context manager, the patching effect is limited to the indented block of code under the with
statement. This is useful when you want to apply the patch to a specific portion of your code. Here is an example of using patch()
as a context manager:
from unittest.mock import patch
def test_function():
with patch('module_name.object_to_patch') as mock_object:
mock_object.return_value = 'mocked_value'
# Code where the patch is active
In the example above, patch()
is used as a context manager to temporarily replace object_to_patch
from module_name
with mock_object
inside the with
block.
Example Usage
Let’s consider a scenario where you want to test a function that makes a request to an external API using the requests
module. You can use patch()
as a context manager to mock the requests.get()
method and simulate a timeout. Here's how you can achieve this:
# my_calendar.py
import requests
def get_calendar_events():
response = requests.get('https://api.example.com/events')
return response.json()
# test_my_calendar.py
import unittest
from unittest.mock import patch
from my_calendar import get_calendar_events
class TestCalendar(unittest.TestCase):
def test_get_calendar_events_timeout(self):
with patch('my_calendar.requests.get') as mocked_requests:
mocked_requests.side_effect = Timeout
result = get_calendar_events()
self.assertEqual(result, [])
In this example, the requests.get()
method is temporarily replaced with a mock object mocked_requests
inside the with
block. The side_effect
is set to Timeout
to simulate a timeout scenario. This allows you to test the behavior of get_calendar_events()
under a timeout condition without actually making a network request.
Conclusion
Using patch()
as a context manager provides a flexible way to manage the scope of patching within your test cases. It allows you to isolate the effects of patching to specific sections of your code, making it a powerful tool for testing in Python.
In this tutorial, you learned how to use patch()
as a context manager in Python for mocking objects during testing. This technique can be valuable when testing code that interacts with external dependencies, such as APIs, databases, or third-party libraries.
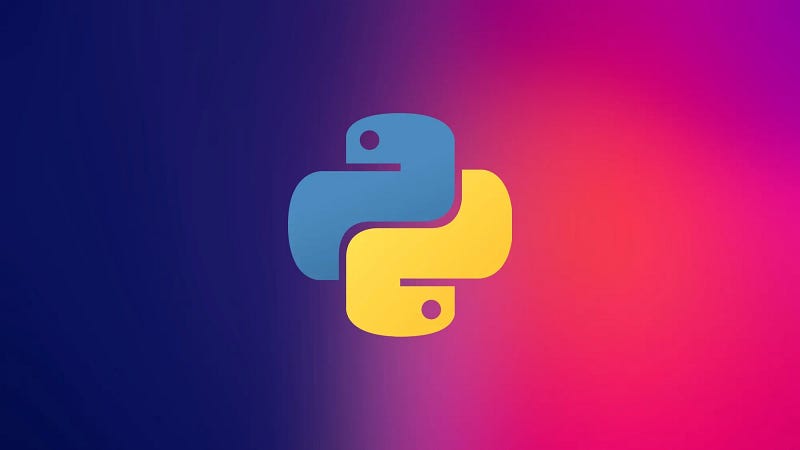