Python Iterators and Iterables Explained with Code Examples
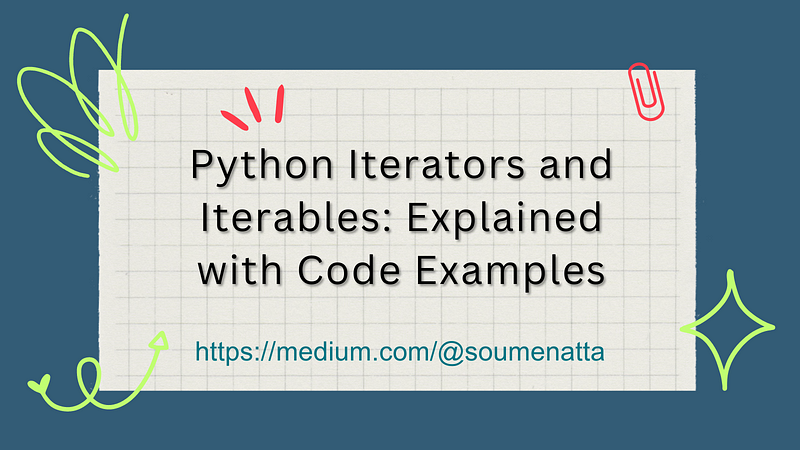
Iterators and iterables are fundamental concepts in Python that facilitate efficient looping and data traversal. This tutorial will provide you with a clear understanding of iterators and iterables, along with code examples to demonstrate their usage.
Table of Contents
- Introduction to Iterators and Iterables
- Iterables: Objects with
__iter__
Method - Iterators: Objects with
__next__
Method - Using Iterators and Iterables in Loops
- Creating Custom Iterables and Iterators
- Conclusion
1. Introduction to Iterators and Iterables
- Iterables: Objects that can be looped over or iterated upon. Lists, tuples, strings, dictionaries, and more are examples of iterables.
- Iterators: Objects that allow you to iterate through an iterable’s elements. They maintain the state of the iteration.
2. Iterables: Objects with __iter__ Method
Iterables have an __iter__
method, which returns an iterator object. The iterator object is used to traverse the elements.
my_list = [1, 2, 3]
my_iter = my_list.__iter__() # Equivalent to iter(my_list)
print(next(my_iter)) # Output: 1
print(next(my_iter)) # Output: 2
print(next(my_iter)) # Output: 3
3. Iterators: Objects with __next__ Method
Iterators have a __next__
method that provides the next element in the sequence. When no more elements are left, it raises the StopIteration
exception.
my_list = [1, 2, 3]
my_iter = iter(my_list)
print(my_iter.__next__()) # Output: 1
print(my_iter.__next__()) # Output: 2
print(my_iter.__next__()) # Output: 3
4. Using Iterators and Iterables in Loops
Python’s for
loop is designed to work seamlessly with iterables. When looping, it internally handles creating an iterator and advancing through the elements.
my_list = [1, 2, 3]
for item in my_list:
print(item) # Output: 1 2 3
5. Creating Custom Iterables and Iterators
You can create your own custom iterables and iterators by implementing the __iter__
and __next__
methods.
class PowerOfTwo:
def __init__(self, max_power):
self.max_power = max_power
self.current_power = 0
def __iter__(self):
return self
def __next__(self):
if self.current_power <= self.max_power:
result = 2 ** self.current_power
self.current_power += 1
return result
else:
raise StopIteration
powers = PowerOfTwo(4)
for val in powers:
print(val) # Output: 1 2 4 8 16
6. Conclusion
In this tutorial, you’ve learned about the fundamental concepts of iterators and iterables in Python. Iterables are objects that can be looped over, while iterators are objects that maintain the state of the iteration. You’ve seen how to create and use both built-in and custom iterables and iterators to traverse data efficiently.
By mastering iterators and iterables, you’ll be able to take full advantage of Python’s looping capabilities and create more efficient and expressive code. These concepts are crucial for effective data manipulation, and they underlie many Python programming paradigms.