Neural Network Training with Convolutional Neural Net (CNN)
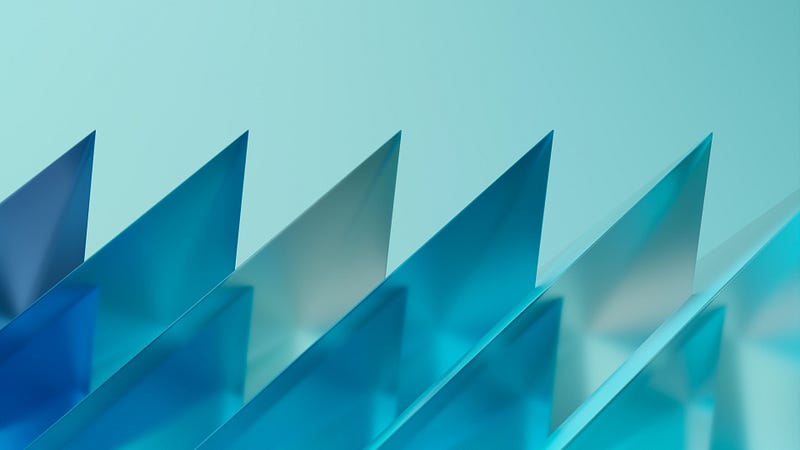
Convolutional Neural Networks (CNNs) are a class of deep neural networks designed for tasks involving grid-structured data, such as images and video. CNNs have proven highly effective in computer vision tasks, achieving state-of-the-art performance in tasks like image classification, object detection, and image segmentation. Here’s an overview of key concepts related to CNNs:
I. Convolutional Neural Network Architect:
Let’s illustrate the architecture of a Convolutional Neural Network (CNN).
Input Image
↓
----------------
| Conv Layer |
----------------
↓
----------------
| Pooling |
----------------
↓
________________
| |
| Conv Layer |
|________________|
↓
----------------
| Pooling |
----------------
↓
----------------
| Conv Layer |
----------------
↓
________________
| |
| Fully |
| Connected |
| Layer |
|________________|
↓
----------------
| Output |
| Layer |
----------------
- The input is an image, typically represented as a grid of pixel values.
- Convolutional layers consist of filters (kernels) that slide over the input image to extract features. Each filter detects patterns at different spatial hierarchies.
- The output of convolutional layers is called feature maps.
- Pooling layers downsample the spatial dimensions of the feature maps, reducing the amount of information while retaining important features. Common pooling operations include max pooling and average pooling.
- After several convolutional and pooling layers, the high-level reasoning in the neural network is flattened into a vector and passed through one or more fully connected layers.
- The fully connected layers combine the learned features and make final predictions.
- The output layer produces the final predictions based on the learned features. The number of neurons in this layer depends on the task (e.g., binary classification, multi-class classification).
- Activation functions, such as ReLU in the convolutional and fully connected layers, introduce non-linearity into the model.
- This architecture is effective for image-related tasks, as CNNs can automatically learn hierarchical representations of visual patterns. The convolutional and pooling layers capture local patterns, while the fully connected layers aggregate these patterns for higher-level reasoning. CNNs are widely used in image classification, object detection, and other computer vision tasks.
II. Convolutional Layers:
1. Input Data:
- Imagine a 2D input data, such as a grayscale image or a single channel from a colored image.
Input Data:
+---+---+---+---+
| 1 | 2 | 3 | 4 |
+---+---+---+---+
| 5 | 6 | 7 | 8 |
+---+---+---+---+
| 9 |10 |11 |12 |
+---+---+---+---+
|13 |14 |15 |16 |
+---+---+---+---+
2. Learnable Filter (Kernel):
- A small learnable filter or kernel is defined. The size of the filter is typically smaller than the input data.
Filter (3x3):
+---+---+---+
| a | b | c |
+---+---+---+
| d | e | f |
+---+---+---+
| g | h | i |
+---+---+---+
3. Convolution Operation:
- The filter slides (convolves) over the input data, element-wise multiplication is performed, and the results are summed to produce a single value in the output feature map.
- The filter is applied to different positions in the input data, capturing local patterns.
Convolution Result (Output Feature Map):
+---+---+---+
| s1| s2| s3|
+---+---+---+
| s4| s5| s6|
+---+---+---+
| s7| s8| s9|
+---+---+---+
- The values
s1
,s2
, ...,s9
in the output feature map are computed based on the convolution operation at each position.
4. Striding and Padding:
- Striding defines how much the filter moves (steps) across the input data.
- Padding may be applied to the input data to ensure that the filter covers the edges properly.
- The convolution operation allows the network to detect local patterns or features in the input data, enabling the model to learn hierarchical representations. Convolutional layers with multiple filters learn to detect different features, creating a rich set of learned patterns that contribute to the network’s understanding of the input.
5. Example:
from keras.models import Sequential
from keras.layers import Conv2D
# Create a Sequential model
model = Sequential()
# Add a convolutional layer with 32 filters (kernels), each of size 3x3
model.add(Conv2D(32, kernel_size=(3, 3), input_shape=(64, 64, 3), activation='relu'))
III. Activation Function:
1. Convolution Result (Output Feature Map):
- Assume we have an output feature map obtained from a convolution operation:
Convolution Result (Output Feature Map):
+---+---+---+
| s1| s2| s3|
+---+---+---+
| s4| s5| s6|
+---+---+---+
| s7| s8| s9|
+---+---+---+
2. ReLU Activation:
- Commonly ReLU (Rectified Linear Unit) is used after convolutions.
- The ReLU activation function is applied element-wise to each value in the feature map.
- ReLU(x) = max(0, x)
- If the value is positive, it remains unchanged; if it’s negative, it is replaced with zero.
ReLU Activation Result:
+---+---+---+
| s1| s2| s3|
+---+---+---+
| s4| s5| 0|
+---+---+---+
| s7| s8| s9|
+---+---+---+
- The non-linear ReLU activation introduces non-linearity to the model, allowing it to learn complex patterns and relationships.
3. Visualization:
- A visualization of the ReLU activation can be seen as follows, where the negative values are clipped to zero:
ReLU(x):
+---+---+---+
| s1| s2| s3|
+---+---+---+
| s4| s5| 0|
+---+---+---+
| s7| s8| s9|
+---+---+---+
- The ReLU activation function is popular in CNNs due to its simplicity and efficiency. It helps the network learn and propagate gradients during backpropagation, promoting faster and more effective training. The non-linear nature of ReLU also enables the network to model complex relationships in the data.
4. Example Code:
from keras.models import Sequential
from keras.layers import Conv2D, Activation
# Create a Sequential model
model = Sequential()
# Add a convolutional layer with 32 filters and a ReLU activation function
model.add(Conv2D(32, kernel_size=(3, 3), input_shape=(64, 64, 3)))
model.add(Activation('relu'))
III. Pooling Layers:
- Reduces computational complexity.
- Helps make the model more robust to variations in input.
1. Input Feature Map (After Convolution):
- Assume we have an input feature map obtained from a convolutional layer:
Input Feature Map:
+---+---+---+---+
| s1| s2| s3| s4|
+---+---+---+---+
| s5| s6| s7| s8|
+---+---+---+---+
| s9|s10|s11|s12|
+---+---+---+---+
|s13|s14|s15|s16|
+---+---+---+---+
2. Pooling Operation (Max Pooling):
- Max pooling is a common pooling operation. It involves selecting the maximum value from a group of values in the input feature map.
- Example of max pooling with a 2x2 window and a stride of 2:
Max Pooling Result:
+---+---+
| s6| s8|
+---+---+
|s14|s16|
+---+---+
- For each 2x2 window, the maximum value is selected.
3. Pooling Operation (Average Pooling):
- Average pooling involves taking the average of a group of values in the input feature map.
- Example of average pooling with a 2x2 window and a stride of 2:
Average Pooling Result:
+---+---+
|s3 |s4 |
+---+---+
|s11|s12|
+---+---+
- For each 2x2 window, the average value is computed.
4. Visualization:
- A visualization of the pooling operation, where each 2x2 window results in a single value:
Pooling Result:
+---+---+
| s6| s8|
+---+---+
|s14|s16|
+---+---+
- The pooling layer is often used to downsample the spatial dimensions of the feature map, reducing computational complexity and focusing on the most important features.
- It also helps the network become more invariant to small translations in the input data.
- Max pooling is particularly effective in capturing dominant features, while average pooling can be used for a smoother downsampling effect.
5. Example Code:
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D
# Create a Sequential model
model = Sequential()
# Add a convolutional layer with 32 filters and ReLU activation function
model.add(Conv2D(32, kernel_size=(3, 3), input_shape=(64, 64, 3), activation='relu'))
# Add a max pooling layer with pool size (2, 2)
model.add(MaxPooling2D(pool_size=(2, 2)))
IV. Flattening:
- After convolutional and pooling layers, the high-level reasoning in the neural network is flattened into a vector.
1. Feature Map After Pooling:
- Assume we have a feature map obtained after applying convolutional and pooling layers:
Feature Map:
+---+---+
| s6| s8|
+---+---+
|s14|s16|
+---+---+
2. Flattening Operation:
- Flattening involves converting the two-dimensional feature map into a one-dimensional vector. This is achieved by reshaping the matrix into a single row.
Flattened Vector:
+---+---+---+---+
| s6| s8|s14|s16|
+---+---+---+---+
- The entire content of the feature map is now represented as a linear sequence of values.
3. Visualization:
- A visualization of the flattened vector:
Flattened Vector:
+---+---+---+---+
| s6| s8|s14|s16|
+---+---+---+---+
- The flattening operation is typically applied after the convolutional and pooling layers in a Convolutional Neural Network (CNN).
- It transforms the spatial information captured in the feature map into a format suitable for input to fully connected layers.
- These flattened vectors are then passed through one or more fully connected layers for further processing and eventual output.
4. Code Example:
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D, Flatten
# Create a Sequential model
model = Sequential()
# Add a convolutional layer with 32 filters and ReLU activation function
model.add(Conv2D(32, kernel_size=(3, 3), input_shape=(64, 64, 3), activation='relu'))
# Add a max pooling layer with pool size (2, 2)
model.add(MaxPooling2D(pool_size=(2, 2)))
# Add a flattening layer
model.add(Flatten())
V. Fully Connected (Dense) Layers:
- Dense layers are used for final classification/regression.
- Outputs are often processed by softmax activation for classification tasks.
1. Flattened Vector from Previous Layer:
- Assume we have a flattened vector obtained after the flattening operation:
Flattened Vector:
+---+---+---+---+
| s6| s8|s14|s16|
+---+---+---+---+
2. Fully Connected Layer:
- Each neuron in a fully connected layer is connected to every neuron in the previous layer. These connections are represented by weights.
- For simplicity, let’s consider a fully connected layer with three neurons.
Neuron 1 Neuron 2 Neuron 3
+---+ +---+ +---+
| w1|----(s6)---->| w2|----(s8)---->| w3|
+---+ +---+ +---+
- Each connection is associated with a weight (w1, w2, w3). The output of each neuron is computed as the weighted sum of the inputs, and an activation function (e.g., ReLU or sigmoid) is applied.
3. Activation Function:
- The output of each neuron in the fully connected layer is often passed through an activation function. This introduces non-linearity into the model.
Neuron 1 Neuron 2 Neuron 3
+---+ +---+ +---+
|ReLU| |ReLU| |ReLU|
+---+ +---+ +---+
- In this case, the ReLU activation function is applied element-wise to the outputs.
4. Final Output:
- The output of the fully connected layer is a vector that can be used for various tasks, such as classification or regression.
Fully Connected Layer Output:
+-----+-----+-----+
|out1 |out2 |out3 |
+-----+-----+-----+
- Each element in the output vector corresponds to the activation of a neuron in the fully connected layer.
- Fully Connected Layers are crucial for capturing complex patterns and relationships in the data. They provide a flexible way for the network to learn and combine features from different parts of the input space.
5. Example Code:
- Below is an example code using Keras to build a simple Convolutional Neural Network (CNN) for image classification. This example assumes a binary classification task. Adjust the architecture, parameters, and output layer for your specific problem.
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
# Initialize the CNN
cnn_model = Sequential()
# Step 1: Convolution Layer
cnn_model.add(Conv2D(filters=32, kernel_size=(3, 3), activation='relu', input_shape=(64, 64, 3)))
# Step 2: Pooling Layer
cnn_model.add(MaxPooling2D(pool_size=(2, 2)))
# Adding a second convolutional layer and pooling layer
cnn_model.add(Conv2D(filters=64, kernel_size=(3, 3), activation='relu'))
cnn_model.add(MaxPooling2D(pool_size=(2, 2)))
# Step 3: Flattening
cnn_model.add(Flatten())
# Step 4: Full Connection
cnn_model.add(Dense(units=128, activation='relu'))
cnn_model.add(Dense(units=1, activation='sigmoid'))
# Compile the CNN
cnn_model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Display the summary of the model architecture
cnn_model.summary()
This example assumes:
- Input images are in RGB format with dimensions 64x64 pixels.
- The first convolutional layer has 32 filters, and the second has 64.
- Pooling layers use max-pooling with a pool size of (2, 2).
- The fully connected layer has 128 neurons.
- The output layer uses a sigmoid activation for binary classification.
VI. Architectural Patterns:
- LeNet-5: One of the early CNN architectures designed for handwritten digit recognition.
- AlexNet: Popularized CNNs with a deeper architecture for the ImageNet competition.
- VGGNet: Known for its simplicity with stacking small-sized kernels.
- GoogLeNet (Inception): Introduced the concept of inception modules with parallel convolutions.
- ResNet: Introduced residual connections to address vanishing gradient problems in very deep networks.
VII. Transfer Learning:
- Leveraging pre-trained CNNs for new tasks.
- Common architectures for transfer learning include VGG16, VGG19, ResNet, Inception, etc.
from keras.applications.vgg16 import VGG16
from keras.models import Sequential
from keras.layers import Dense, Flatten
# Load the pre-trained VGG16 model
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
# Create a Sequential model
model = Sequential()
# Add the pre-trained VGG16 model to the new model (excluding the top dense layers)
model.add(base_model)
# Flatten the output of the VGG16 model
model.add(Flatten())
# Add a new dense layer for your specific task (e.g., binary classification)
model.add(Dense(units=256, activation='relu'))
# Add the final output layer
model.add(Dense(units=1, activation='sigmoid'))
VIII. Data Augmentation:
- Techniques to artificially increase the diversity of the training dataset.
- Helps the model generalize better to unseen data.
# Set up data augmentation
datagen = ImageDataGenerator(
rescale=1./255,
rotation_range=20,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
fill_mode='nearest'
)
# Example of loading and augmenting images from a directory
train_generator = datagen.flow_from_directory(
'train_data_directory',
target_size=(64, 64),
batch_size=32,
class_mode='binary'
)
# Train the model using the augmented data generator
model.fit(train_generator, epochs=10)
IX. Object Detection and Localization:
- CNNs are commonly used for object detection tasks.
- Anchor boxes and region proposal networks (RPN) are used in modern architectures.
X. Semantic Segmentation:
- Assigning each pixel in an image to a specific class.
- Often involves encoder-decoder architectures.
XI. Hyperparameter Tuning:
- Choosing the right architecture, learning rate, batch size, etc., is crucial.
- Overfitting is a common concern, and techniques like dropout and regularization are used.
from tensorflow import keras
from tensorflow.keras import layers
from kerastuner.tuners import RandomSearch
# Function to build the model
def build_model(hp):
model = keras.Sequential()
model.add(layers.Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3)))
model.add(layers.MaxPooling2D((2, 2)))
model.add(layers.Flatten())
model.add(layers.Dense(128, activation='relu'))
model.add(layers.Dense(1, activation='sigmoid'))
# Tune the learning rate
hp_learning_rate = hp.Choice('learning_rate', values=[1e-2, 1e-3, 1e-4])
model.compile(optimizer=keras.optimizers.Adam(learning_rate=hp_learning_rate),
loss='binary_crossentropy',
metrics=['accuracy'])
return model
# Instantiate the tuner
tuner = RandomSearch(
build_model,
objective='val_accuracy',
max_trials=5, # Adjust as needed
executions_per_trial=3, # Adjust as needed
directory='hyperparameter_tuning',
project_name='my_cnn_tuning'
)
# Search for the best hyperparameter configuration
tuner.search(train_data, train_labels, epochs=10, validation_data=(val_data, val_labels))
# Get the best model and hyperparameters
best_model = tuner.get_best_models(1)[0]
best_hyperparameters = tuner.get_best_hyperparameters(1)[0]
# Display the best hyperparameters
print(f"Best learning rate: {best_hyperparameters.get('learning_rate')}")
XII. Frameworks:
- Commonly implemented using deep learning frameworks like TensorFlow and PyTorch.
XIII. Challenges:
1. Vanishing Gradient:
- Deep networks suffer from vanishing gradient problems during backpropagation.
2. Overfitting:
- Need for regularization techniques to prevent overfitting, especially with limited data.
3. Computational Complexity:
- Deep networks can be computationally intensive.
Convolutional Neural Networks have played a pivotal role in advancing the field of computer vision, enabling machines to understand and interpret visual information with remarkable accuracy. They continue to be a foundational technology in various applications, from image recognition to medical image analysis.