Interview Questions — Optional in Java
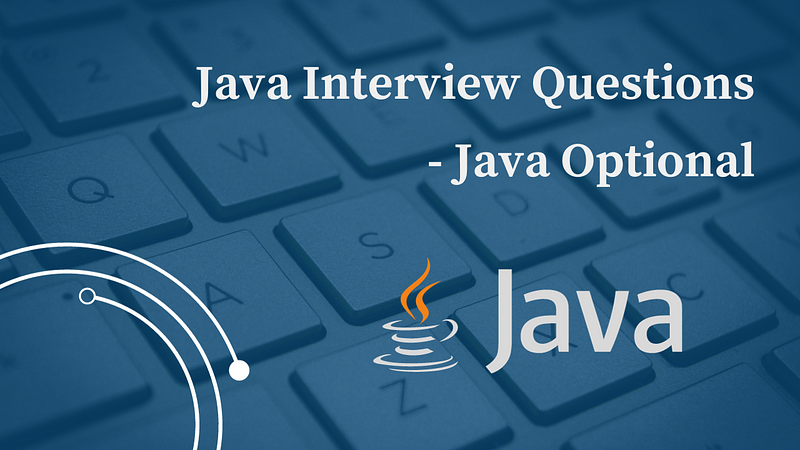
Java introduced the Optional
class in Java 8 as part of the java.util
package. It provides a more eloquent way to deal with cases that may or may not produce a value, thus helping to handle the absence of value situations without null references. Since its inception, Optional
has been frequently used as a return type for methods, emphasizing that the method might not always return a meaningful value.
In this article, we’ll discuss some of the commonly asked interview questions related to Java Optionals, along with their short answers.
If you don’t have a medium membership, you can use this link to reach the article without a paywall.
1. What is Java Optional?
Optional
is a container object that may or may not contain a non-null value. If a value is present, Optional.isPresent()
returns true
and Optional.get()
returns the value. Otherwise, isPresent()
returns false
and get()
throws a NoSuchElementException
.
2. Why was Optional introduced?
Optional
was introduced to:
- Provide a clear and explicit way to signal the possibility of an absent value.
- Help in avoiding null references, thereby reducing the chances of
NullPointerException
. - Offer a more expressive, readable API for dealing with optional values compared to null checks.
3. How do you create an Optional object?
There are several ways:
Optional.of(T value)
: Returns anOptional
with the specified non-null value.Optional.ofNullable(T value)
: Returns anOptional
with the specified value, or an emptyOptional
if the value is null.Optional.empty()
: Returns an emptyOptional
.
4. What is the purpose of the isPresent() method in Optional?
The isPresent()
method is used to check whether an Optional
instance contains a value or is empty. It returns true
if a value is present and false
if the Optional
is empty.
Optional<String> optional = Optional.of("Hello, World!");
if (optional.isPresent()) {
// Optional has a value.Do something with the value
}
5. How do you retrieve the value inside an Optional?
You can use the get()
method, but you should ensure the Optional
contains a value by using isPresent()
or otherwise, it will throw a NoSuchElementException
. Alternatively, you can use methods like orElse()
, orElseGet()
, and orElseThrow()
to provide default values or actions in case the Optional
is empty.
6. What are the differences between orElse() and orElseGet()?
Both methods provide a way to retrieve the value from the Optional
or a default value if the Optional
is empty:
orElse(T other)
: Returns the value if present; otherwise, it returns theother
value. The argument is evaluated even if theOptional
has a value.orElseGet(Supplier<? extends T> other)
: Returns the value if present; otherwise, it returns the result produced by the supplier. The supplier is only invoked if theOptional
is empty.
7. How can you transform the value inside an Optional?
You can use the map()
method. It applies the given function to the value, then wraps the result in an Optional
.
8. How do you handle situations where you have a function that returns an Optional, and you want to apply it to a value inside another Optional?
You can use the flatMap()
method. Unlike map()
, flatMap()
is used when the function returns an Optional
. It flattens two nested Optional
objects into one.
map
and flatMap
are methods in Optional
for transforming the contained value while preserving the optionality. Here’s the difference between them:
map(Function<? super T,? extends U> mapper)
: This method applies the provided function to the value inside theOptional
if it’s present and returns a newOptional
containing the result. It allows you to transform the value without unwrapping it.
Optional<String> optional = Optional.of("Hello, World!");
Optional<Integer> lengthOptional = optional.map(String::length);
flatMap(Function<? super T,Optional<U>> mapper)
: This method is similar tomap
, but the provided function returns anotherOptional
. It then flattens the result into a singleOptional
. It’s useful when you have a function that returns anOptional
, and you want to combine multiple optionals into one.
Optional<String> optional = Optional.of("Hello, World!");
Optional<Integer> lengthOptional = optional.flatMap(val -> Optional.of(val.length()));
Using map
and flatMap
allows you to perform transformations on Optional
values in a more functional and concise manner.
9. How can you filter an Optional based on a condition?
You can use the filter
method to conditionally filter an Optional
and retain the value if it meets a certain condition. If the condition is not met, it returns an empty Optional
.
Optional<String> optional = Optional.of("Hello, World!");
Optional<String> filtered = optional.filter(val -> val.contains("Hello,"));
In this example, filtered
will contain the value if it contains "Hello," otherwise, it will be empty.
10. Can Optional be used with Java Streams?
Yes, Optional
can be combined with streams. For instance, methods like map()
, flatMap()
, and filter()
in Optional
have similar semantics to their counterparts in the Stream API. Moreover, you can convert an Optional
to a stream using the stream()
method introduced in Java 9.
11. When would you use Optional as a return type in a method?
Optional
is often used as a return type in methods when the result may or may not be present, and it's not appropriate to return null
. It makes it explicit in the method signature that the result is optional and forces the calling code to handle both cases (presence and absence of a value).
public Optional<String> findValueById(int id) {
// Implement logic to find a value by ID
// Return Optional.of(value) if found, Optional.empty() if not
}
This allows callers to use methods like ifPresent
, orElse
, or orElseGet
to handle the result gracefully.
12. Is it recommended to use Optional for the arguments or fields of a class?
While Optional
is great for return types, it's not recommended to use it as a field type or method parameter type. Using it in such places can make the code overly verbose and might confuse other developers.
Conclusion
Java’s Optional
class is a powerful tool for enhancing code readability and preventing potential pitfalls related to null values. Knowing how to effectively use and understand Optional
is a valuable skill for any Java developer, especially when navigating the modern Java ecosystem. By understanding the intricacies of Optional
, you are better prepared for both real-world coding and interview scenarios.
👏 Thank You for Reading!
👨💼 I appreciate your time and hope you found this story insightful. If you enjoyed it, don’t forget to show your appreciation by clapping 👏 for the hard work and subscribe
📰 Keep the Knowledge Flowing by Sharing the Article!
✍ Feel free to share your feedback or opinions about the story. Your input helps me improve and create more valuable content for you.
✌ Stay Connected! 🚀 For more engaging articles, make sure to follow me on social media:
🔍 Explore More! 📖 Dive into a treasure trove of knowledge at Codimis. There’s always more to learn, and we’re here to help you on your journey of discovery.