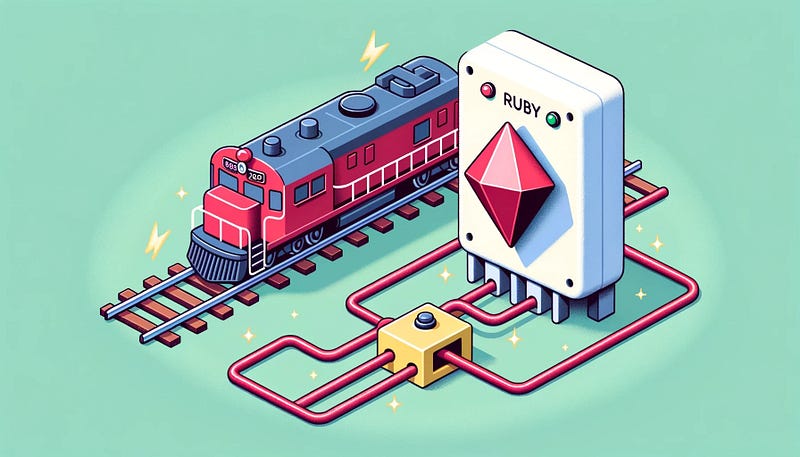
Implementing a Circuit Breaker in Rails
The Circuit Breaker pattern is a design pattern used in software development to improve the stability and resilience of a system. In a Rails application, using a circuit breaker can prevent your system from making unnecessary requests to external services when they are known to be failing. This pattern can be particularly useful when your Rails application interacts with external APIs, databases, or other microservices.
The Basics of the Circuit Breaker Pattern
Think of a circuit breaker in electrical systems. It trips or “breaks” the circuit when there’s too much current, preventing potential damage. Similarly, in software, a circuit breaker “trips” when certain conditions are met, usually after detecting that a particular service is unavailable or failing.
Here are the three primary states of a circuit breaker:
- Closed: Everything is functioning as expected. Requests are made to the external service.
- Open: The external service is down or unresponsive. Requests are not made; instead, failures are returned immediately.
- Half-Open: After a predetermined amount of time, the circuit breaker allows some requests to check if the service has recovered. If it’s stable, the state returns to Closed; otherwise, it remains Open.
Implementing a Simple Circuit Breaker in Rails
Let’s design a simple circuit breaker without relying on any gems or plugins.
Model the Circuit Breaker
class CircuitBreaker
attr_accessor :failure_count, :state, :last_failed_time
FAILURE_THRESHOLD = 5
HALF_OPEN_TIME = 60 # seconds
def initialize
@failure_count = 0
@state = :closed
@last_failed_time = nil
end
def request
case state
when :closed
make_request
when :open
raise "Service Unavailable"
when :half_open
make_request if Time.now - last_failed_time >= HALF_OPEN_TIME
end
end
private
def make_request
# Replace with your actual request to an external service
response = ExternalService.call
if response.success?
reset
else
register_failure
end
rescue
register_failure
raise "Service Error"
end
def register_failure
@failure_count += 1
@last_failed_time = Time.now
if failure_count >= FAILURE_THRESHOLD
@state = :open
end
end
def reset
@state = :closed
@failure_count = 0
end
end
Using the Circuit Breaker
Whenever you want to make a request to the external service, you would call the request
method on an instance of CircuitBreaker
.
cb = CircuitBreaker.new
cb.request
Enhancements
While this is a basic implementation, in real-world scenarios, you may want to:
- Store the state of the circuit breaker in a distributed cache like Redis if you have multiple instances of your application.
- Add logging to monitor and alert when the circuit is open.
- Make the thresholds and timings configurable.
Conclusion
The Circuit Breaker pattern provides an elegant solution to handle failures and improve the resilience of your Rails application. With this simple implementation, you can begin to protect your application from cascading failures and ensure a better experience for your users.