Exploring Map() vs. Starmap() in Python
Let’s learn about the differences
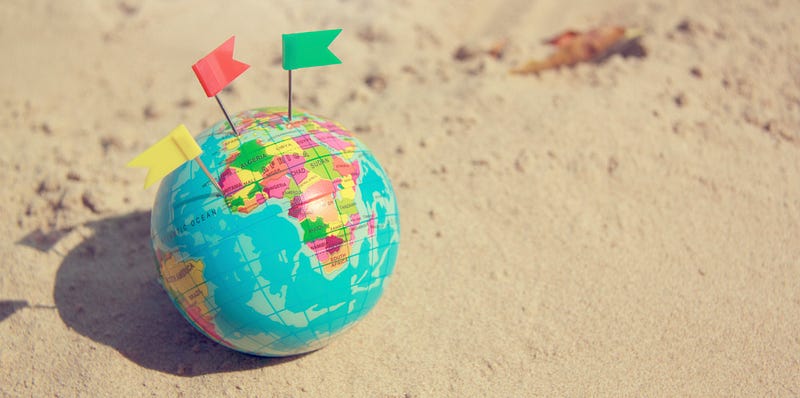
map
“
map(function, iterable, ...)
Return an iterator that applies a function to every item of iterable, yielding the results. If additional iterable arguments are passed, the function must take that many arguments and is applied to the items from all iterables in parallel. With multiple iterables, the iterator stops when the shortest iterable is exhausted.” — Python’s documentation
- The
map()
function is used to apply a function to each item in the iterable. - We can also pass multiple iterables, but the function mentioned should also have that many arguments (e.g. two iterables means two arguments).
- If multiple iterables given are of different lengths, the
map
iterator stops when the shortest iterable is exhausted. - The return type is a
map
object. - A
map
object is an iterator.

We can access the map
object, which is an iterator, in the following ways:
- We can convert the map object to sequence objects like a list using a
list()
constructor and like a tuple using atuple()
constructor. - We can also iterate through the
map
object using afor
loop. - We can access the element in the
map
object using thenext()
function as well.
Example 1: Applying a function to all items in one iterable using map()
- The
square()
function is defined to return the square the numbers. - In the
map
function, we are passing thesquare()
function and list object. - The
square()
function is applied to all items in the list object. - The return type is a
map
object. - A
map
object is an iterator that contains the square of all items in the iterable (list object). - Convert the
map
object to a list using alist()
constructor.