Angular 17 Routing: Enhancing Modern Web Applications
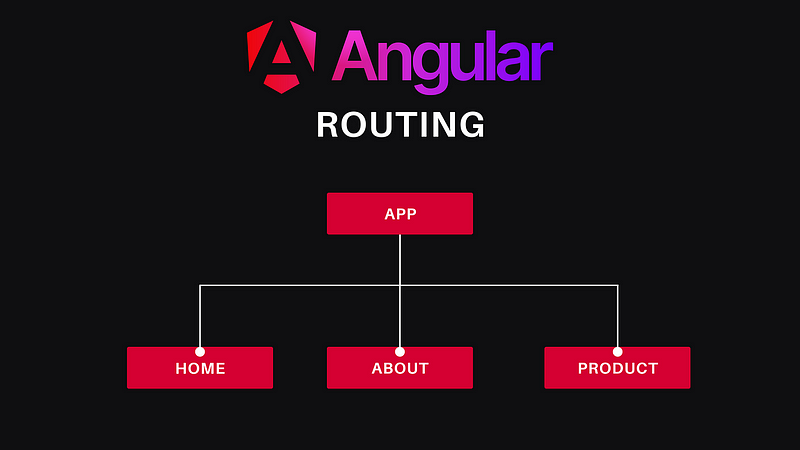
Angular 17 has advanced its routing capabilities to enable seamless and efficient navigation in modern web applications. This article explores the core aspects of Angular routing and how to effectively implement it in projects.
Fundamentals of Angular Routing
What is Routing in Angular?
Routing in Angular facilitates navigation between different views within a Single Page Application (SPA) without reloading the page. It leverages browser history to simulate the user experience of a Multi-Page Application while remaining a single page.
Configuring Routes
Configuring Routes in Angular 17
In Angular 17, route configuration has been refined. Routes are now defined in a separate file, typically named app.routes.ts
, which is a departure from the previous convention where routes were often defined within the AppModule or a dedicated AppRoutingModule. This change promotes a clearer separation of concerns and improves modularity.
import { Routes } from '@angular/router';
export const routes: Routes = [
{ path: 'home', component: HomeComponent },
{ path: 'about', component: AboutComponent },
// other routes...
];
Router Outlet
`<router-outlet>` acts as a dynamic placeholder in the App component, rendering the component of the current route. When a route is activated, Angular loads the corresponding component into the `<router-outlet>` element.
<router-outlet></router-outlet>
Navigation
Navigation can be achieved in two ways:
- Declaratively: Using the `routerLink` directive in HTML templates.
<a routerLink="/home">Home</a>
<a routerLink="/about">About</a>
2. Programmatically: Through the Router service in TypeScript.
navigateToHome() {
this.router.navigate(['/home']);
}
Advanced Routing Concepts
Lazy Loading
Lazy loading is a technique where features are encapsulated in separate modules and loaded only when needed, reducing the initial load time of the application.
{ path: 'feature', loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule) }
Route Guards
Route guards are services that control routing based on certain conditions. The most common guards are:
- CanActivate: Determines if a route can be activated.
- CanDeactivate: Determines if navigation away from a route can occur.
- Resolve: Fetches data before a route is activated.
Path Matching Strategies
- full: Matches only if the entire URL path matches the route path.
- prefix: Matches if the beginning of the URL path matches the route path.
Full Matching Strategy
The full
strategy requires that the entire URL path matches exactly the path of the route. This is useful for avoiding accidental matches with routes that have similar starting paths but are meant to lead to different components.
Example:
Consider an application with two routes, one for a welcome page and another for a user profile:
const routes: Routes = [
{ path: 'welcome', component: WelcomeComponent, pathMatch: 'full' },
{ path: 'user/profile', component: UserProfileComponent }
];
In this case, navigating to /welcome
would load the WelcomeComponent
because it matches the full path. However, navigating to /welcome/info
would not match this route and would look for other routes that match.
Prefix Matching Strategy
The prefix
strategy matches if the beginning of the URL path matches the route path. This allows for more flexible routing structures, especially when dealing with nested routes or modules.
Example:
Consider an application that has a base route for user-related features and nested routes for specific user actions:
const routes: Routes = [
{ path: 'user', component: UserComponent, children: [
{ path: 'profile', component: UserProfileComponent },
{ path: 'settings', component: UserSettingsComponent }
]},
// other routes...
];
Here, the base path /user
uses a prefix strategy by default. Navigating to /user/profile
or /user/settings
would match the base path and then use the child routes to load the respective components. The URL just needs to start with /user
to match the route, making it more versatile for matching nested paths.
Practical Use Cases
- Full: Use the
full
strategy for top-level routes or when you have distinct paths that should not be confused with one another. It ensures a strict match and is clear about what URL loads what component. - Prefix: The
prefix
strategy is ideal for modular applications or when you have a set of related routes under a common path segment. It provides the flexibility to expand your application with nested routes without changing the existing URL structure.
Parameterized Routes
Parameterized routes allow embedding variables in routes, useful for retrieving detail views or performing actions based on IDs.
{ path: 'user/:id', component: UserComponent }
In the `UserComponent`, parameters can be retrieved using the `ActivatedRoute` service.
Redirections and Wildcard Routes
Redirections are used to redirect users to another route. Wildcard routes catch invalid URLs.
{ path: '', redirectTo: '/home', pathMatch: 'full' },
{ path: '**', component: NotFoundComponent }
Best Practices
- Modularity: Organize routes in feature modules to improve maintainability and clarity.
- Guard Services: Use guard services for authentication and authorization.
- Lazy Loading: Leverage lazy loading to enhance your application’s efficiency.
- Data Resolution: Use resolvers to load data before activating a route.
Conclusion
Routing in Angular 17 offers a powerful platform for creating SPAs with seamless transitions between views. By understanding and applying the concepts and best practices presented, developers can implement effective and user-friendly navigation systems in their applications.
Thank you for exploring Angular 17’s routing capabilities with me. This framework’s evolution continues to offer developers powerful tools for building efficient, user-friendly single-page applications. For more discussions on Angular and web development, visit my website:
Let’s keep pushing the boundaries of what we can create with technology, sharing knowledge and insights along the way.